Java IO: DataOutputStream
Jakob Jenkov |
The Java DataOutputStream
class enables you to write Java primitives to OutputStream
's instead
of only bytes. You wrap an OutputStream
in a DataOutputStream
and then you can write primitives
to it. That is why it is called a DataOutputStream - because you can write int
, long
,
float
and double
values to the OutputStream
, and not just raw bytes.
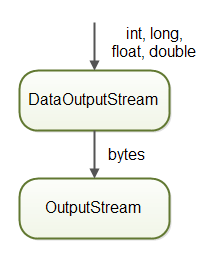
Often you will use the Java DataOutputStream
together with a Java DataInputStream
.
You use the DataOutputStream
to write the data to e.g. a file, and then use the DataInputStream
to read the data again. I have included an example of that later.
Using the DataOutputStream
and DataInputStream
together is a handy way to be able
to write larger primitives than bytes to an OutputStream
and be able to read them in again, ensuring
the right byte order is used etc.
DataOutputStream Example
Here is a Java DataOutputStream
example:
DataOutputStream dataOutputStream = new DataOutputStream( new FileOutputStream("binary.data")); dataOutputStream.write(45); //byte data dataOutputStream.writeInt(4545); //int data dataOutputStream.writeDouble(109.123); //double data dataOutputStream.close();
This example first creates a DataOutputStream
which writes the converted data to a FileOutputStream
.
Second, the example writes a byte
, an int
and a double
to the
DataOutputStream
which converts these primitives to bytes and writes them to the FileOutputStream
.
In the end the DataOutputStream
is closed.
Using a DataOutputStream With a DataInputStream
As mentioned earlier, the DataOutputStream
class is often used together with a DataInputStream
.
Therefore I just want to show you an example of first writing data with a DataOutputStream
and
then reading it again with a DataInputStream
. Here is the example Java code:
import java.io.*; public class DataOutputStreamExample { public static void main(String[] args) throws IOException { DataOutputStream dataOutputStream = new DataOutputStream( new FileOutputStream("data/data.bin")); dataOutputStream.writeInt(123); dataOutputStream.writeFloat(123.45F); dataOutputStream.writeLong(789); dataOutputStream.close(); DataInputStream dataInputStream = new DataInputStream( new FileInputStream("data/data.bin")); int int123 = dataInputStream.readInt(); float float12345 = dataInputStream.readFloat(); long long789 = dataInputStream.readLong(); dataInputStream.close(); System.out.println("int123 = " + int123); System.out.println("float12345 = " + float12345); System.out.println("long789 = " + long789); } }
This example first creates a DataOutputStream
and then writes an int
, float
and a long
value to a file. Second the example creates a DataInputStream
which reads
the int
, float
and long
value in from the same file.
Closing a DataOutputStream
When you are finished writing data to the DataOutputStream
you should remember to close it.
Closing a DataOutputStream
will also close the OutputStream
instance to which the
DataOutputStream
is writing.
Closing a DataOutputStream
is done by calling its close()
method. Here is how
closing a DataOutputStream
looks:
dataOutputStream.close();
You can also use the try-with-resources construct
introduced in Java 7. Here is how to use and close a DataOutputStream
looks with the try-with-resources
construct:
OutputStream output = new FileOutputStream("data/data.bin"); try(DataOutputStream dataOutputStream = new DataOutputStream(output)){ dataOutputStream.writeInt(123); dataOutputStream.writeFloat(123.45F); dataOutputStream.writeLong(789); }
Notice how there is no longer any explicit close()
method call. The try-with-resources construct
takes care of that.
Notice also that the first FileOutputStream
instance is not created inside
the try-with-resources block. That means that the try-with-resources block will not automatically close this
FileOutputStream
instance. However, when the DataOutputStream
is closed
it will also close the OutputStream
instance it writes to, so the FileOutputStream
instance will get closed when the DataOutputStream
is closed.
Tweet | |
Jakob Jenkov |