Java Collections
Jakob Jenkov |
The Java Collections API provide Java developers with a set of classes and interfaces that makes it easier to work with collections of objects, e.g. lists, maps, stacks etc.
Rather than having to write your own collection classes, Java provides these ready-to-use collection classes for you. This tutorial will look closer at the Java Collections, as they are also sometimes referred to, and more specifically the Java Collections available in Java 8 and later.
The purpose of this tutorial is to give you an overview of the Java Collection classes. Thus it will not describe each and every little detail of the Java Collection classes. But, once you have an overview of what is there, it is much easier to read the rest in the JavaDoc afterwards.
Java Collections Tutorial Video
If you prefer learning via video, I have a playlist of Java collections tutorial videos covering some
of the core classes and interfaces in the Java Collections API:
Java Collections Tutorial Video Playlist
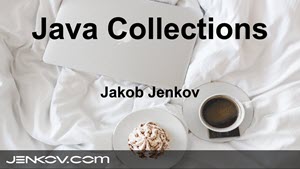
Java Collection Core Classes and Interfaces
The core interfaces of the Java Collection API are:
- Java Collection
- Java List
- Java Set
- Java SortedSet
- Java navigableSet
- Java Map
- Java SortedMap
- Java NavigableMap
- Java Stack
- Java Queue
- Java Deque
- Java Iterator
- Java Iterable
Most of these core interfaces are explained in more detail in their own tutorials, but I will give you a quick introduction in the following sections.
The Java Collections API also contains useful implementations of the above interfaces, and a few other utility classes. Some of these are:
Java Collection
The Java Collection interface represents the operations possible on a generic collection, like on a List, Set, Stack, Queue and Deque. For instance, methods to access the elements based on their index are available in the Java Collection interface. The Java Collection interface is explained in more detail in the Java Collection interface tutorial.
Java List
The Java List interface represents an ordered collection of objects. By ordered means, that you can access the elements in the order they occur in the list. The List interface is explained in more detail in the Java List Interface tutorial.
Java Set
The Java Set interface represents an unordered collection of objects. Unlike the List, a Set does not allow you to access the elements of a Set in any guaranteed order. There are Set implementations that order elements based on their natural ordering, but the Set interface itself provides no such guarantees. The Set interface is explained in more detail in the Java Set tutorial.
Java SortedSet
The Java SortedSet interface represents an ordered collection of objects. Thus, the elements in the SortedSet can be iterated in the sorted order. The SortedSet interface is explained in more detail in the Java SortedSet tutorial.
Java NavigableSet
The Java NavigableSet interface is an extension of the SortedSet interface with additional methods for eay navigation of the elements in the NavigableSet. The SortedSet interface is explained in more detail in the Java NavigableSet tutorial.
Java Map
The Java Map interface represents a mapping between sets of keys and values. Both keys and values are objects. You insert a key + value pair into a Map, and later you can retrieve the value via the key - meaning you only need the key to read the value out of the Map again later. The Map interface is explained in more detail in the Java Map tutorial.
Java SortedMap
The Java SortedMap interface is an extension of the Map interface, representing a Map where the keys in the Map are sorted. Thus, you can iterate the keys stored in the SortedMap in the sorted order, rather than the kind-of-random order you iterate them in a normal Map. The SortedMap interface is explained in more detail in the Java SortedMap tutorial.
Java NavigableMap
The Java NavigableMap interface is an extension of the SortedMap interface which contains additional methods for easy navigation of the keys and entries in the NavigableMap. The NavigableMap interface is explained in more detail in the Java NavigableMap tutorial.
Java Stack
The Java Stack class represents a classical stack data structure, where elements can be pushed to the top of the stack, and popped off from the top of the stack again later. The Stack interface is explained in more detail in the Java Stack tutorial.
Java Queue
The Java Queue interface represents a classical queue data structure, where objects are inserted into one end of the queue, and taken off the queue in the other end of the queue. This is the opposite of how you use a stack. The Queue interface is explained in more detail in the Java Queue tutorial.
Java Deque
The Java Deque interface represents a double ended queue, meaning a data structure where you can insert and remove elements from both ends of the queue. I guess you could have called it a double ended stack too. The deque interface is explained in more detail in the Java Deque tutorial.
Java Iterator
The Java Iterator interface represents a component that is capable of iterating a Java collection of some kind. For instance, a List or a Set. You can obtain an Iterator instance from the Java Set, List, Map etc.
Java Iterable
The Java Iterable interface is very similar in responsibility to the Java Iterator interface. The Iterable interface allows a Java Collection to be iterated using the for-each loop in Java. The Java Iterable interface is explained in more detail in my tutorial about the Java Iterable interface tutorial. The Java Iterable interface is not actually a part of the Java Collection API, but it is used so often with the Java Collection API that I have included the tutorial as part of the Java Collection tutorial. The for-each loop is explained in my Java for Loop tutorial.
Java Collections Class
The Java Collections class contains a range of utility methods that help you work more efficiently with the Java Collections API.
Java Properties
The Java Properties class is a special key-value store similar to a Java Map, but specifically targeted at keeping string-string key-value pairs, and being able to load and store properties from property files.
Java Collection Packages
Most of the Java collections are located in the java.util
package. Java
also has a set of concurrent collections in the java.util.concurrent
package. This tutorial will not describe the concurrent collections. These are
described in my Java util concurrent tutorial.
Overview of Java Collections
To help you get an overview of the Java Collections classes and interfaces, the first text in this Java Collections tutorial is the Java Collections Overview text.
Java Collections and Generics
The fifth text in this Java Collections tutorial covers how to use Generics in Java Collections. Generics is very useful when working with Java's Collection classes. You can find the tutorial at Java Generic Collections.
Java Generics in general is explained in more detail in my Java Generics tutorial.
Java Collections and the equals() and hashCode() Methods
Many of the core components in the Java Collection API rely on the correct implementation of the
hashCode()
and equals()
methods. The Java hashCode and equals methods
tutorial explain how these methods work, and why they are central to the core Java collection components.
Tweet | |
Jakob Jenkov |