JavaFX ScatterChart
Jakob Jenkov |
The JavaFX ScatterChart component can draw scatter charts inside your JavaFX applications. The
JavaFX ScatterChart component is represented by the class javafx.scene.chart.ScatterChart
.
ScatterChart X Axis and Y Axis
A JavaFX ScatterChart draws a scatter chart. A scatter chart is a two-dimensional graph, meaning the graph has an X axis
and a Y axis. You can use both categorical and numerical axes, but in this example I will just use two numerical
axes for the scatter chart. A numerical axis is represented by the JavaFX class javafx.scene.chart.NumberAxis
.
You need to define the X axis and Y axis used by a ScatterChart
.
Here is an example of creating two JavaFX NumberAxis
instances:
NumberAxis xAxis = new NumberAxis(); xAxis.setLabel("No of employees"); NumberAxis yAxis = new NumberAxis(); yAxis.setLabel("Revenue per employee");
Creating a ScatterChart
You create a JavaFX ScatterChart component by creating an instance of the ScatterChart
class.
You need to pass an X axis and Y axis to the ScatterChart
constructor. Here is a
JavaFX ScatterChart
instantiation example:
NumberAxis xAxis = new NumberAxis(); xAxis.setLabel("No of employees"); NumberAxis yAxis = new NumberAxis(); yAxis.setLabel("Revenue per employee"); ScatterChart scatterChart = new ScatterChart(xAxis, yAxis);
ScatterChart Data Series
To get a JavaFX ScatterChart
component to display any dots, you must provide it with a data series.
A data series is a list of data points. Each data point contains an X value and a Y value. Here is an example
of creating a data series and adding it to a ScatterChart
component:
XYChart.Series dataSeries1 = new XYChart.Series(); dataSeries1.setName("2014"); dataSeries1.getData().add(new XYChart.Data( 1, 567)); dataSeries1.getData().add(new XYChart.Data( 5, 612)); dataSeries1.getData().add(new XYChart.Data(10, 800)); dataSeries1.getData().add(new XYChart.Data(20, 780)); dataSeries1.getData().add(new XYChart.Data(40, 810)); dataSeries1.getData().add(new XYChart.Data(80, 850)); scatterChart.getData().add(dataSeries1);
First an XYChart.Series
instance is created and given a name.
Second, 6 XYChart.Data
instances are added to the XYChart.Series
object.
Third, the XYChart.Series
object is added to a ScatterChart
object.
It is possible to add multiple data series to the ScatterChart
. Just repeat the above code
for additional data series.
Adding a ScatterChart to the Scene Graph
To make a ScatterChart
visible you must add it to the JavaFX scene graph. This means adding the
ScatterChart
to a Scene
object or add the ScatterChart
to a layout
component which is added to a Scene
object.
Here is an example that adds a ScatterChart
to the JavaFX scene graph:
package com.jenkov.javafx.charts; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.chart.NumberAxis; import javafx.scene.chart.ScatterChart; import javafx.scene.chart.XYChart; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class ScatterChartExperiments extends Application { @Override public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("BarChart Experiments"); NumberAxis xAxis = new NumberAxis(); xAxis.setLabel("No of employees"); NumberAxis yAxis = new NumberAxis(); yAxis.setLabel("Revenue per employee"); ScatterChart scatterChart = new ScatterChart(xAxis, yAxis); XYChart.Series dataSeries1 = new XYChart.Series(); dataSeries1.setName("2014"); dataSeries1.getData().add(new XYChart.Data( 1, 567)); dataSeries1.getData().add(new XYChart.Data( 5, 612)); dataSeries1.getData().add(new XYChart.Data(10, 800)); dataSeries1.getData().add(new XYChart.Data(20, 780)); dataSeries1.getData().add(new XYChart.Data(40, 810)); dataSeries1.getData().add(new XYChart.Data(80, 850)); scatterChart.getData().add(dataSeries1); VBox vbox = new VBox(scatterChart); Scene scene = new Scene(vbox, 400, 200); primaryStage.setScene(scene); primaryStage.setHeight(300); primaryStage.setWidth(1200); primaryStage.show(); } public static void main(String[] args) { Application.launch(args); } }
The application resulting from running this application would look similar to this:
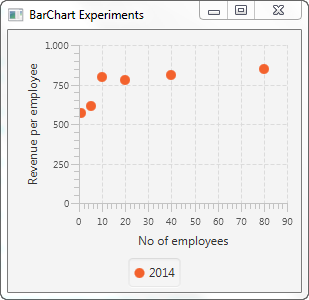
Tweet | |
Jakob Jenkov |