JavaFX Slider
Jakob Jenkov |
The JavaFX Slider control provides a way for the user to select a value within a given interval
by sliding a handle to the desired point representing the desired value. The JavaFX Slider
is represented by the JavaFX class javafx.scene.control.Slider
. Here is a screenshot of
how a JavaFX Slider
looks:
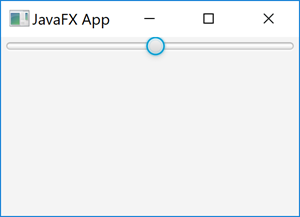
JavaFX Slider Example
Here is a full JavaFX Slider
code example:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Slider; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class SliderExample extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { primaryStage.setTitle("JavaFX App"); Slider slider = new Slider(0, 100, 0); VBox vBox = new VBox(slider); Scene scene = new Scene(vBox, 960, 600); primaryStage.setScene(scene); primaryStage.show(); } }
Create a Slider
To use a JavaFX Slider
you must first create an instance of the Slider
class.
Here is an example of creating a JavaFX Slider
instance:
Slider slider = new Slider(0, 100, 0);
The Slider
constructor used above takes three parameters: The min value, the max value and the initial value.
The min value is the value sliding the handle all the way to the left represents. This is the beginning of the interval
the user can select a value in. The max value is the value sliding the handle all the way to the right represents.
This the end of the interval the user can select a value in. The initial value is the value that the handle should
be located at, when presented to the user at first.
Reading Slider Value
You can read the value of a Slider
as selected by the user via the getValue()
method.
Here is an example of reading the selected value of a JavaFX Slider
:
double value = slider.getValue();
Major Tick Unit
You can set the major tick unit of a JavaFX Slider
control. The major tick unit is
how many units the value changes every time the user moves the handle of the Slider
one tick.
Here is an example that sets the major tick unit of a JavaFX Slider
to 8:
Slider slider = new Slider(0, 100, 0); slider.setMajorTickUnit(8.0);
This Slider
will have its value change with 8.0 up or down whenever the handle in the Slider
is moved.
Minor Tick Count
You can set the minor tick count of a JavaFX Slider
via the setMinorTickCount()
method. The minor tick count specifies how many minor ticks there are between two of the major ticks.
Here is an example that sets the minor tick count to 2:
Slider slider = new Slider(0, 100, 0); slider.setMajorTickUnit(8.0); slider.setMinorTickCount(3);
The Slider
configured here has 8.0 value units between each major tick, and in between each of
these major ticks it has 3 minor ticks.
Snap Handle to Ticks
You can make the handle of the JavaFX Slider
snap to the ticks using the Slider
setSnapToTicks()
method, passing a parameter value of true
it. Here is an example
of making the JavaFX Slider
snap its handle to the ticks:
slider.setSnapToTicks(true);
Show Tick Marks
You can make the JavaFX Slider show marks for the ticks when it renders the slider.
You do so using its setShowTickMarks()
method. Here is an example of making a JavaFX Slider
show tick marks:
slider.setShowTickMarks(true);
Here is a screenshot of how a JavaFX Slider
looks with tick marks shown:
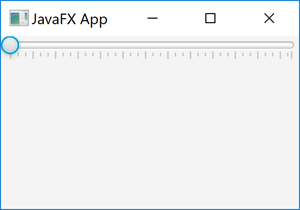
Show Tick Labels
You can make the JavaFX Slider
show tick labels for the ticks when it renders the slider.
You do so using its setShowTickLabels()
method. Here is an example of making a JavaFX Slider
show tick labels:
slider.setShowTickLabels(true);
Here is a screenshot of how a JavaFX Slider
looks with tick marks and labels shown:
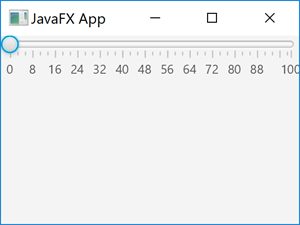
Tweet | |
Jakob Jenkov |