JavaFX ToggleButton
Jakob Jenkov |
A JavaFX ToggleButton is a button that can be selected or not selected. Like a button that stays in when you
press it, and when you press it the next time it comes out again. Toggled - not toggled. The JavaFX ToggleButton
is represented by the class javafx.scene.control.ToggleButton
.
Creating a ToggleButton
You create a JavaFX ToggleButton by creating an instance of the ToggleButton
class. Here is
an example of creating a JavaFX ToggleButton
instance:
ToggleButton toggleButton1 = new ToggleButton("Left");
This example creates a ToggleButton
with the text Left
on.
Adding a ToggleButton to the Scene Graph
To make a ToggleButton
visible you must add it to the JavaFX scene graph. This means adding it to
a Scene
, or as child of a layout which is attached to a Scene
object.
Here is an example that attaches a JavaFX ToggleButton
to the scene graph:
package com.jenkov.javafx.controls; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.ToggleButton; import javafx.scene.layout.HBox; import javafx.stage.Stage; public class ToggleButtonExperiments extends Application { @Override public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("HBox Experiment 1"); ToggleButton toggleButton1 = new ToggleButton("Left"); HBox hbox = new HBox(toggleButton1); Scene scene = new Scene(hbox, 200, 100); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { Application.launch(args); } }
The application resulting from running the above example code is illustrated in the following two screenshots.
The first screenshot shows a ToggleButton
which is not pressed, and the second screenshot shows
the same ToggleButton
pressed (selected, activated etc.):
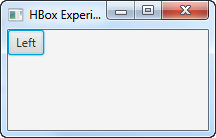
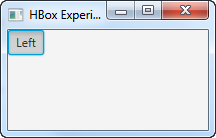
ToggleButton Text
You can set or change the text of a JavaFX ToggleButton via its setText()
method. Here is an example of
changing the text of a JavaFX ToggleButton via setText()
:
ToggleButton toggleButton = new ToggleButton("Toggle This!"); toggleButton.setText("New Text");
ToggleButton Font
You can set the font to use to render the button text of a JavaFX ToggleButton via its setFont()
method. You can read more about
creating fonts in my JavaFX Fonts tutorial. Here is an example of setting the font
of a JavaFX ToggleButton:
ToggleButton toggleButton = new ToggleButton("Toggle This!"); Font arialFontBold36 = Font.font("Arial", FontWeight.BOLD, 36); toggleButton.setFont(arialFontBold36);
Reading Selected State
The ToggleButton
class has a method named isSelected
which lets you determine if the
ToggleButton
is selected (pressed) or not. The isSelected()
method returns a boolean
with the value true
if the ToggleButton
is selected, and false
if not.
Here is an example:
boolean isSelected = toggleButton1.isSelected();
ToggleGroup
You can group JavaFX ToggleButton
instances into a ToggleGroup
. A ToggleGroup
allows at most one ToggleButton
to be toggled (pressed) at any time. The ToggleButton
instances in a ToggleGroup
thus functions similarly to radio buttons.
Here is a JavaFX ToggleGroup
example:
ToggleButton toggleButton1 = new ToggleButton("Left"); ToggleButton toggleButton2 = new ToggleButton("Right"); ToggleButton toggleButton3 = new ToggleButton("Up"); ToggleButton toggleButton4 = new ToggleButton("Down"); ToggleGroup toggleGroup = new ToggleGroup(); toggleButton1.setToggleGroup(toggleGroup); toggleButton2.setToggleGroup(toggleGroup); toggleButton3.setToggleGroup(toggleGroup); toggleButton4.setToggleGroup(toggleGroup);
Here is a full example that adds the 4 ToggleButton
instances to a ToggleGroup
, and
adds them to the scene graph too:
package com.jenkov.javafx.controls; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.ToggleButton; import javafx.scene.control.ToggleGroup; import javafx.scene.layout.HBox; import javafx.stage.Stage; public class ToggleButtonExperiments extends Application { @Override public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("HBox Experiment 1"); ToggleButton toggleButton1 = new ToggleButton("Left"); ToggleButton toggleButton2 = new ToggleButton("Right"); ToggleButton toggleButton3 = new ToggleButton("Up"); ToggleButton toggleButton4 = new ToggleButton("Down"); ToggleGroup toggleGroup = new ToggleGroup(); toggleButton1.setToggleGroup(toggleGroup); toggleButton2.setToggleGroup(toggleGroup); toggleButton3.setToggleGroup(toggleGroup); toggleButton4.setToggleGroup(toggleGroup); HBox hbox = new HBox(toggleButton1, toggleButton2, toggleButton3, toggleButton4); Scene scene = new Scene(hbox, 200, 100); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { Application.launch(args); } }
The resulting applications looks like this:
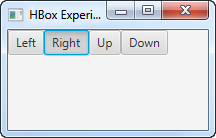
Reading Selected State of a ToggleGroup
You can read which ToggleButton
of a ToggleGroup
is selected (pressed) using the
getSelectedToggle()
method, like this:
ToggleButton selectedToggleButton = (ToggleButton) toggleGroup.getSelectedToggle();
If no ToggleButton
is selected the getSelectedToggle()
method returns null
.
Tweet | |
Jakob Jenkov |